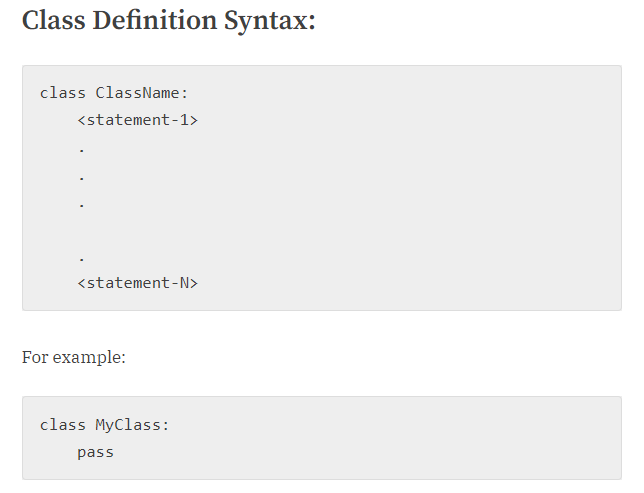
Creating a Class in Python
In this article, we will explore the process of creating a class in Python, discuss its benefits, and provide examples to help you understand this fundamental concept.
Understanding the concept of classes in Python
Object-oriented programming (OOP) is a programming paradigm that organizes code by bundling related properties and behaviours into individual objects.
In Python, OOP is widely used, and it revolves around the concept of classes and objects. A class is a blueprint or prototype from which objects are created. It encapsulates attributes (data) and methods (functions) that define the behavior of the objects.
By creating a class, we can define a specific type of object with its own unique characteristics and functionalities. This allows for code reusability and modularity, making it easier to manage and maintain complex programs.
Basic Syntax and Structure of a Class in Python
To create a class in Python, we use the keyword “class” followed by the name of the class. The basic syntax and structure of a class include defining attributes and methods. Attributes are variables that store data specific to each instance of the class, while methods are functions that define the behaviour of the class.
Class Definition Syntax:
class ClassName:
<statement-1>
.
.
.
.
<statement-N>
For example:
class MyClass:
pass
In the example above, we define a class named MyClass
with no attributes or methods. The pass
statement is a placeholder that allows the class to be defined without any content. However, a class without any attributes or methods serves no practical purpose.
Creating a Class and Defining Attributes
Methods are defined within the class using the “def” keyword, and they typically have the first parameter named “self”. The “self” parameter refers to the instance of the class and allows access to its attributes and methods.
By creating a class, we can create multiple instances or objects of that class, each with its own unique set of attribute values. Classes provide a means of bundling data and functionality together, promoting code organization and reusability.
In Python, almost everything is an object, and classes serve as blueprints for creating objects with specific properties and behaviors. Understanding and utilizing classes in Python is essential for building complex and scalable programs. In the following sections, we will explore different aspects of classes and how they can be effectively used in Python programming.
Why Should You Use Classes?
Classes play a crucial role in object-oriented programming (OOP) and offer several benefits:
- Code Organization: Classes enable you to group related variables and functions together, making your code more organized and easier to maintain.
- Code Reusability: By creating classes, you can reuse code across different parts of your program or even in other projects.
- Modularity: Classes allow you to break down complex problems into smaller, more manageable components, promoting modularity and scalability.
- Abstraction: Classes provide a level of abstraction by hiding the internal details of an object and exposing only the necessary interface.
- Inheritance: Inheritance allows you to create new classes based on existing ones, inheriting their attributes and methods. This promotes code reuse and enhances flexibility.
Creating an Instance of a Class
To use a class and access its attributes and methods, you need to create an instance of the class. An instance, also known as an object, is a specific occurrence of a class.
my_object = MyClass()
In the code snippet above, we create an instance of the MyClass
class and assign it to the variable my_object
. Now, my_object
represents an object with the attributes and methods defined in the class.
Class Attributes vs. Instance Attributes
In Python, classes can have two types of attributes: class attributes and instance attributes.
- Class Attributes: Class attributes are shared by all instances of a class. They are defined within the class but outside any method.
- Instance Attributes: Instance attributes are unique to each instance of a class. They are defined within the methods of the class using the
self
keyword.
class MyClass:
class_attribute = "This is a class attribute"
def __init__(self):
self.instance_attribute = "This is an instance attribute"
In the example above, class_attribute
is a class attribute, while instance_attribute
is an instance attribute. Class attributes are accessed using the class name, whereas instance attributes are accessed using the instance name.
Class Methods vs. Instance Methods
In addition to attributes, classes can also have methods. Methods are functions defined within a class and are used to perform specific actions on the objects of the class.
- Class Methods: Class methods are defined using the
@
classmethod
decorator and have access to the class itself, rather than individual instances. They can be used to modify class attributes or perform operations that are not specific to any particular instance. - Instance Methods: Instance methods are defined without any decorators and operate on individual instances of a class. They have access to instance attributes and can modify them accordingly.
class MyClass:
class_attribute = "This is a class attribute"
def instance_method(self):
# Access instance attributes and perform actions
pass
@classmethod
def class_method(cls):
# Access class attributes and perform actions
pass
In the example above, instance_method
is an instance method, and class_method
is a class method.
Inheritance: Extending Classes
Inheritance is a fundamental concept in OOP that allows you to create new classes based on existing ones. The new classes, called derived classes or subclasses, inherit the attributes and methods of the base class or superclass.
class Animal:
def sound(self):
print("The animal makes a sound.")
class Dog(Animal):
def sound(self):
print("The dog barks.")
class Cat(Animal):
def sound(self):
print("The cat meows.")
In the example above, Animal
is the base class, while Dog
and Cat
are derived classes. Both Dog
and Cat
inherit the sound
method from Animal
but can override it with their own implementations.
Overriding Methods in Derived Classes
When a derived class defines a method with the same name as a method in the base class, it is said to override the base class method. This allows the derived class to provide its own implementation of the method.
class Animal:
def sound(self):
print("The animal makes a sound.")
class Dog(Animal):
def sound(self):
print("The dog barks loudly!")
In the example above, the Dog
class overrides the sound
method inherited from Animal
and provides a custom implementation.
Abstract Base Classes (ABCs)
Abstract Base Classes (ABCs) are classes that cannot be instantiated directly but can be used to define a common interface for multiple subclasses. ABCs allow you to define abstract methods that must be implemented by all concrete subclasses.
from abc import ABC, abstractmethod
class Shape(ABC):
@abstractmethod
def area(self):
pass
class Rectangle(Shape):
def area(self):
return self.length * self.width
In the example above, Shape
is an ABC that defines an abstract method area()
. The Rectangle
class is a concrete subclass of Shape
and must implement the area()
method.
Magic Methods in Python Classes
Magic methods, also known as dunder methods, are special methods in Python classes that allow you to define behaviors for built-in operations. These methods have double underscores (__
) at the beginning and end of their names.
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __add__(self, other):
return Vector(self.x + other.x, self.y + other.y)
def __str__(self):
return f"Vector({self.x}, {self.y})"
In the example above, the __add__
method enables the addition of two Vector
objects using the +
operator. The __str__
method defines a string representation for the Vector
class.
Static Methods in Python Classes
Static methods are methods that belong to a class rather than an instance. They do not have access to instance or class attributes and are primarily used for utility functions that are related to the class.
class MathUtils:
@staticmethod
def square(x):
return x ** 2
In the example above, the square
method is a static method defined in the MathUtils
class. It can be called using the class name without creating an instance.
Encapsulation: Access Modifiers
Python does not provide built-in access modifiers like some other languages. However, you can use naming conventions to indicate the intended visibility of attributes and methods.
- Public: Public attributes and methods have no special naming convention and can be accessed from anywhere.
- Protected: Protected attributes and methods are indicated by a single leading underscore (
_
) and should be considered internal to the class or its subclasses. - Private: Private attributes and methods are indicated by a double leading underscore (
__
). They are intended for internal use within the class and are not accessible outside of it.
class MyClass:
def __init__(self):
self.public_attribute = "This is a public attribute"
self._protected_attribute = "This is a protected attribute"
self.__private_attribute = "This is a private attribute"
In the example above, public_attribute
is public, _protected_attribute
is protected, and __private_attribute
is private.
Polymorphism: Using Multiple Classes
Polymorphism is the ability of objects of different classes to respond to the same method. In Python, polymorphism is achieved through method overriding and duck typing.
class Animal:
def sound(self):
pass
class Dog(Animal):
def sound(self):
print("The dog barks.")
class Cat(Animal):
def sound(self):
print("The cat meows.")
def make_sound(animal):
animal.sound()
dog = Dog()
cat = Cat()
make_sound(dog) # Output: The dog barks.
make_sound(cat) # Output: The cat meows.
In the example above, the make_sound
function can accept both Dog
and Cat
objects as arguments because they both respond to the sound
method.
Working with Modules and Classes
Python allows you to organize your code into modules, which are separate files containing Python definitions and statements. Classes can be defined in their own modules and imported into other modules for use.
# file: mymodule.py
class MyClass:
pass
# file: main.py
from mymodule import MyClass
obj = MyClass()
In the example above, the MyClass
class is defined in the mymodule.py
module and imported into the main.py
module for creating instances.
Error Handling in Classes
Error handling is an essential aspect of writing robust and reliable code. In Python classes, you can use try-except
blocks to handle exceptions and gracefully handle errors.
class FileHandler:
def __init__(self, filename):
try:
self.file = open(filename, "r")
except FileNotFoundError:
print("File not found!")
def read_file(self):
try:
return self.file.read()
except AttributeError:
print("No file opened!")
def close_file(self):
try:
self.file.close()
except AttributeError:
print("No file opened!")
In the example above, the FileHandler
class uses try-except
blocks to handle potential exceptions when opening, reading, or closing a file.
Using Decorators in Classes
Decorators are a powerful feature of Python that allow you to modify the behavior of functions or classes. They can be used to add functionality, implement caching, or enforce certain conditions.
def log_calls(func):
def wrapper(*args, **kwargs):
print(f"Calling function: {func.__name__}")
return func(*args, **kwargs)
return wrapper
@log_calls
def greet(name):
print(f"Hello, {name}!")
greet("Alice") # Output: Calling function: greet, Hello, Alice!
In the example above, the log_calls
decorator adds logging functionality to the greet
function, printing the function name before executing it.
Serializing Objects: Pickle Module
Serialization is the process of converting an object into a format that can be stored or transmitted. In Python, the pickle
module provides a convenient way to serialize and deserialize objects.
import pickle
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
person = Person("Alice", 25)
# Serialize the object
serialized = pickle.dumps(person)
# Deserialize the object
deserialized = pickle.loads(serialized)
In the example above, we define a Person
class and use the pickle
module to serialize an instance of the class (person
) into a byte stream and then deserialize it back into an object.
Common Issue and Best Practices
When creating classes in Python, it’s essential to be aware of some common issue and follow best practices to write clean and maintainable code:
- Naming Conventions: Follow the recommended naming conventions to make your code more readable and consistent.
- Class Documentation: Provide clear and informative docstrings for your classes and methods to facilitate understanding and usage.
- Avoid Global State: Minimize the use of global variables and strive for encapsulation and modularity.
- Code Readability: Write code that is easy to understand, follow, and debug by adopting a clear and consistent coding style.
- Code Reusability: Design your classes with reusability in mind, making them modular and decoupled from specific implementations.
- Error Handling: Implement proper error handling and exception handling to handle unexpected situations gracefully.
- Testing: Write unit tests to verify the correctness of your classes and ensure their robustness.
Frequently Asked Questions
Q: What is the difference between a class and an object in Python?
A: A class is a blueprint or template for creating objects, while an object is a specific instance of a class.
Q: Can a class in Python have multiple constructors?
A: No, Python does not support multiple constructors in the same way as some other programming languages. However, you can achieve similar behavior using class methods or optional arguments.
Q: What is the purpose of the self
keyword in Python classes?
A: The self
keyword is a convention in Python that represents the instance of a class. It is used to access instance attributes and methods within the class.
Q: How can I access class attributes in Python?
A: Class attributes can be accessed using the class name followed by the attribute name, for example, ClassName.attribute
.
Q: Can a class in Python inherit from multiple base classes?
A: Yes, Python supports multiple inheritance, allowing a class to inherit attributes and methods from multiple base classes.
Q: What are metaclasses in Python?
A: Metaclasses are classes that define the behavior of other classes. They allow you to customize the creation and behavior of classes.
Conclusion
In this article, we explored the concept of creating a class in Python and discussed its benefits in software development. We covered various aspects of classes, including attributes, methods, inheritance, encapsulation, polymorphism, and more. By understanding and leveraging classes, you can write more organized, reusable, and maintainable code.
Remember to follow best practices, write clear and concise code, and continuously expand your knowledge of Python classes.