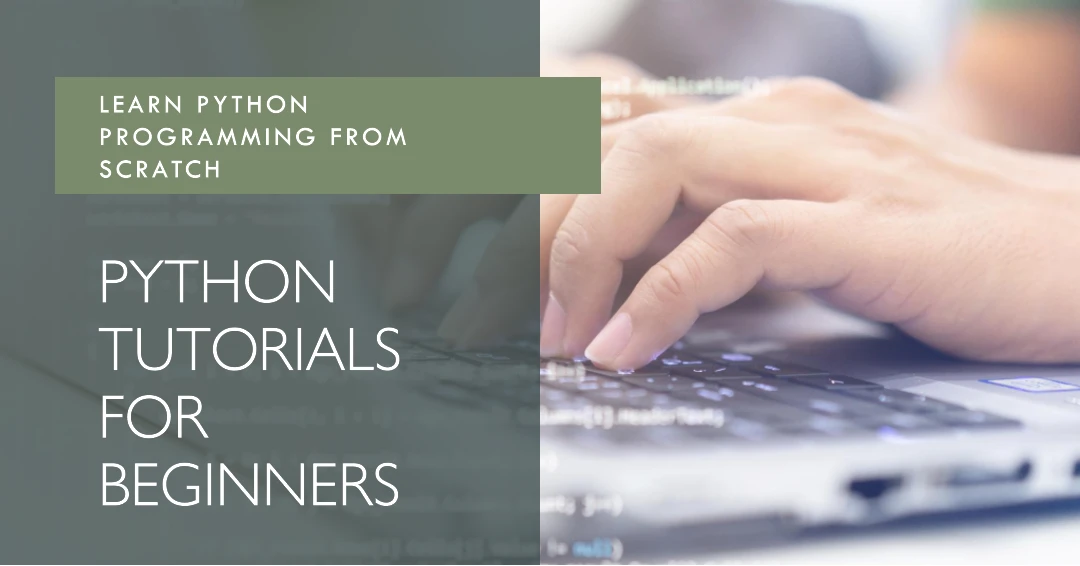
Python Tutorials for Beginners Programmers
In this tutorial, we’ll cover the basics of Python programming tutorials for beginners programmers, including setting up your environment, writing your first program, and exploring the language’s features.
If you are a beginner programmer looking to learn a powerful, versatile programming language, Python is an excellent choice. Python has a simple syntax, making it easy to learn, yet it is also powerful enough to handle complex tasks.
Additionally, Python is widely used in a variety of industries, including web development, data analysis, and artificial intelligence.
Getting Started with Python
Before we dive into the specifics of Python programming, let’s talk about getting set up. The first step in learning Python is to install it on your computer. or you can also start with pythonide.online
Installing Python on Windows & macOS
Python is available for a variety of platforms, including Windows, MacOS, and Linux. Here’s how to get started on the two most popular operating systems:
Installing Python on Windows
To install Python on Windows, follow these steps:
- Visit the Python downloads page at https://www.python.org/downloads/windows/.
- Click the “Download Python” button for the latest version of Python 3.
- Run the downloaded installer and follow the on-screen instructions.
- Once the installation is complete, open a command prompt and type “python” to launch the Python interpreter.
Installing Python on MacOS
To install Python on MacOS, follow these steps:
- Open a web browser and visit https://www.python.org/downloads/macos/.
- Click the “Download Python” button for the latest version of Python 3.
- Run the downloaded installer and follow the on-screen instructions.
- Once the installation is complete, open a Terminal window and type “python” to launch the Python interpreter.
Writing Your First Python Program
Now that you have Python installed, it’s time to write your first program. Open your favorite text editor and create a new file called “hello.py“. In this file, type the following code:
print("Hello, world!")
Save the file and then open a command prompt or Terminal window. Navigate to the directory where you saved the “hello.py” file and then run the following command:
python hello.py
You should see the message “Hello, world!” printed to the console. Congratulations, you’ve written your first Python program!
Here are the Python Data Types
Python supports a variety of data types, including strings, numbers, lists, tuples, and dictionaries.
Strings
Strings are sequences of characters. In Python, you can create a string by enclosing text in either single or double quotes. For example:
name = "John"
Numbers
Python supports two types of numbers: integers and floating-point numbers. Integers are whole numbers, while floating-point numbers have decimal places. For example:
age = 25
height = 1.75
Lists
Lists are collections of items, which can be of different data types. In Python, you can create a list by enclosing items in square brackets, separated by commas. For example:
fruits = ["apple", "banana", "orange"]
You can access individual items in a list by their index, starting at 0. For example, to access the first item in the list above (which is “apple”), you would use the following code:
print(fruits[0])
Tuples
Tuples are similar to lists, but they are immutable, which means their contents cannot be changed after they are created. In Python, you can create a tuple by enclosing items in parentheses, separated by commas. For example:
dimensions = (1920, 1080)
Dictionaries
Dictionaries are collections of key-value pairs. In Python, you can create a dictionary by enclosing key-value pairs in curly braces, separated by commas. For example:
person = {"name": "John", "age": 25}
You can access individual values in a dictionary by their keys. For example, to access the value of the “name” key in the dictionary above (which is “John”), you would use the following code:
print(person["name"])
Control Flow Statements in Python
Control flow statements are used to control the flow of execution in a Python program.
If Statements
If statements are used to execute code only if a certain condition is met. For example:
age = 25
if age >= 18:
print("You are an adult")else:
print("You are a minor")
This code will print “You are an adult” because the age variable is greater than or equal to 18.
While Loops
While loops are used to execute a block of code repeatedly as long as a certain condition is true. For example:
count = 0
while count < 5:
print(count)
count += 1
This code will print the numbers 0 to 4, one on each line.
For Loops
For loops are used to iterate over a collection of items, such as a list or a dictionary. For example:
fruits = ["apple", "banana", "orange"]
for fruit in fruits:
print(fruit)
This code will print each item in the list of fruit on a separate line.
Functions in Python
Functions are reusable blocks of code that perform a specific task.
Defining Functions
To define a function in Python, you use the def keyword, followed by the function name and any arguments the function takes. For example:
def say_hello(name):
print("Hello, " + name)
This code defines a function called say_hello that takes one argument, name. When called, the function will print the message “Hello, ” followed by the value of the name argument.
Function Arguments
Functions can take any number of arguments, which can be of any data type. For example:
def add_numbers(a, b):
return a + b
This code defines a function called add_numbers that takes two arguments, a and b. When called, the function will return the sum of a and b
Function Return Values
Functions can also return a value, which can be of any data type. For example:
def add_numbers(a, b):
return a + b
result = add_numbers(2, 3)print(result)
This code will call the add_numbers function with arguments 2 and 3, which will return the value 5. The value 5 will then be stored in the result variable, and printed to the console.
These are some Python Tutorials for Beginners Programmers
If you are a beginner programmer who is interested in learning Python, there are many resources available to you. Here are a few Python tutorials for beginners that you may find helpful:
Python.org
Python.org is the official website for the Python programming language. It offers a wealth of resources for beginners, including tutorials, documentation, and a community forum where you can ask questions and get help.
Codecademy
Codecademy is an online learning platform that offers a Python course for beginners. The course covers the basics of Python programming, including variables, data types, control flow statements, functions, and more. It also includes interactive exercises and quizzes to help you test your understanding.
Coursera
Coursera is an online learning platform that offers a variety of Python courses for beginners. Some of the courses are free, while others require a fee. The courses cover a range of topics, from basic programming concepts to more advanced topics like machine learning and data analysis.
Udemy
Udemy is an online learning platform that offers a variety of Python tutorials for beginners programmers. Some of the courses are free, while others require a fee. The courses cover a range of topics, from basic programming concepts to more advanced topics like web development and data science.
Conclusion
Python is a powerful and versatile programming language that is easy to learn, making it a great choice for beginner programmers. Whether you are interested in building web applications, data analysis, or machine learning, Python has something to offer. By taking advantage of the many resources available to you, such as Python.org, Codecademy, Coursera, and Udemy, you can quickly get up to speed on the basics of Python programming and start building your own projects.